Is Your LLM API ready for real-world load?
Large Language Models (LLMs) are transforming how we build software—powering everything from chatbots to code generation. But behind the scenes, these AI-powered APIs are resource-intensive, latency-sensitive, and critical to user experience.
If your application depends on an LLM API—whether self-hosted or from a provider like OpenAI—you need to answer a tough question:
👉 Can it scale when real users start hitting it… at full speed?
LLM APIs aren’t like regular APIs
Most APIs deal in milliseconds. LLMs often operate in seconds. Every prompt involves complex computation, model inference, and memory allocation.
That creates unique challenges for engineering teams:
- High response times by default
- Significant infrastructure impact (GPU load, memory spikes, inference cost)
- Unpredictable usage patterns that make peak traffic hard to simulate
Traditional API tests simply aren’t enough. Functional tests won’t catch issues like:
- How many prompts your infrastructure can handle simultaneously
- How latency behaves under load
- When performance drops or errors begin to spike
Skipping load testing for an LLM API isn’t just risky—it’s expensive.
LLM Performance = User Experience + Cost Control
Poor LLM performance doesn’t just frustrate users—it eats into your margins.
If you’re building a customer-facing AI feature, performance lag or failure means:
- ❌ Lower retention and trust
- ❌ Higher support costs
- ❌ Missed SLAs or contractual penalties
For internal tools or developer-facing APIs, the stakes are just as high:
⚠️ Slow APIs break workflows. Unpredictable load kills confidence.
And when every request hits an expensive GPU-backed service, even small inefficiencies turn into big cloud bills.
Bottom line? Load testing your LLM API = better UX, tighter cost control, and fewer surprises in production.
Load Test LLM APIs with Gatling
Gatling makes it easy to simulate realistic LLM traffic at scale—without overcomplicating your test setup.
✅ Test-as-code flexibility
Write test scenarios in JavaScript, TypeScript, Scala, Java, or Kotlin—just like your app. Add loops, delays, and conditionals to mimic actual user interactions with prompts.
✅ Real-world concurrency simulation
Simulate hundreds or thousands of concurrent users sending diverse prompts. Control pacing, request size, and even streaming endpoints.
✅ Insightful performance dashboards
Monitor latency distribution, error spikes, and resource bottlenecks in real time. Compare test runs, track regressions, and optimize before users ever see an issue.
✅ Scale safely with quota & cost controls
Set limits, monitor usage, and avoid runaway tests that burn through tokens or infrastructure credits.
Whether you’re validating a self-hosted LLM stack or stress-testing OpenAI’s API, Gatling helps you move from “We hope it holds” to “We know it scales.”
📘 Ready to stress test your LLM-powered app?
Follow our complete guide to load testing LLM APIs with Gatling:
Share this
You May Also Like
These Related Articles
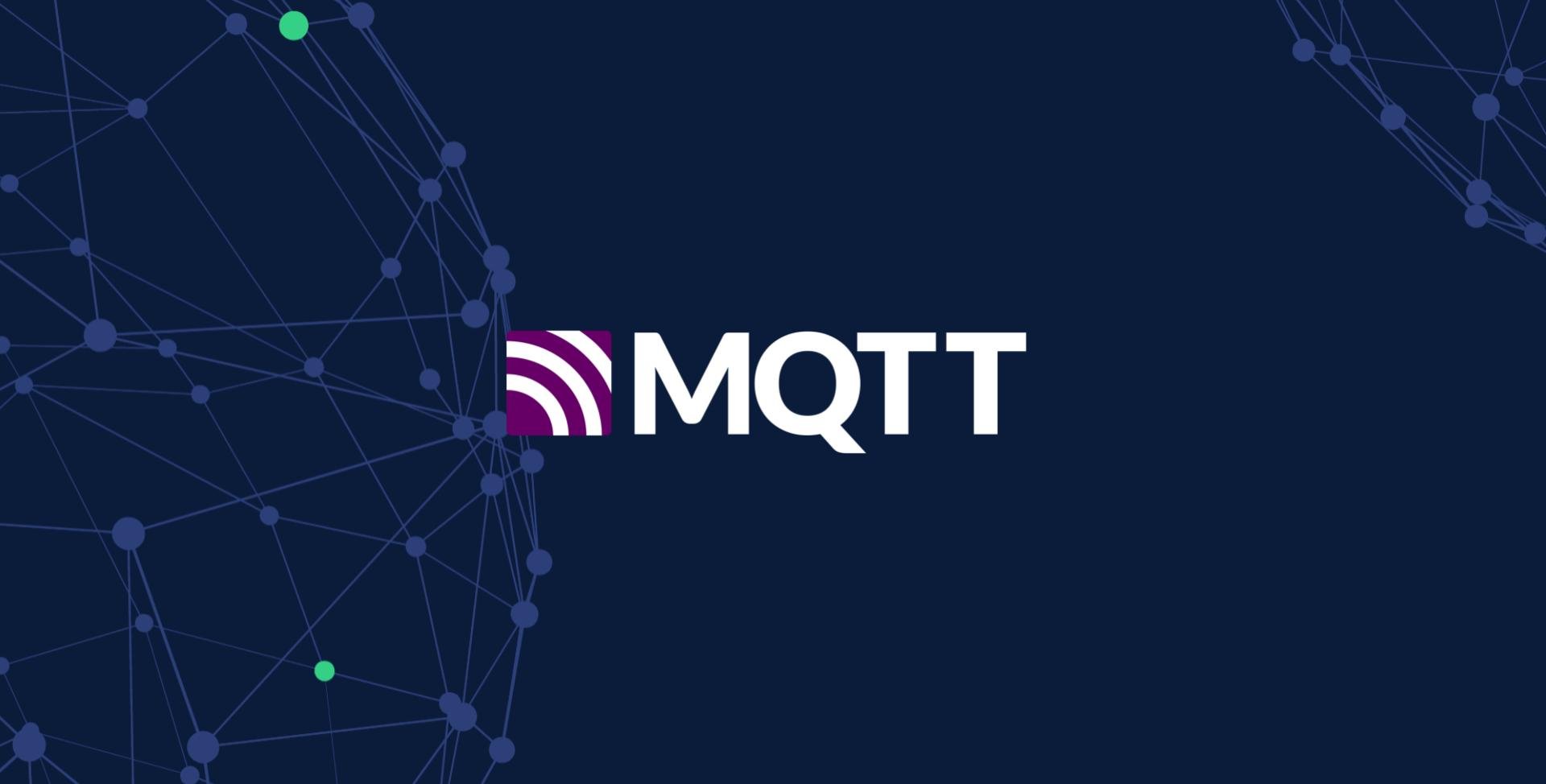
How to load test MQTT applications with Gatling
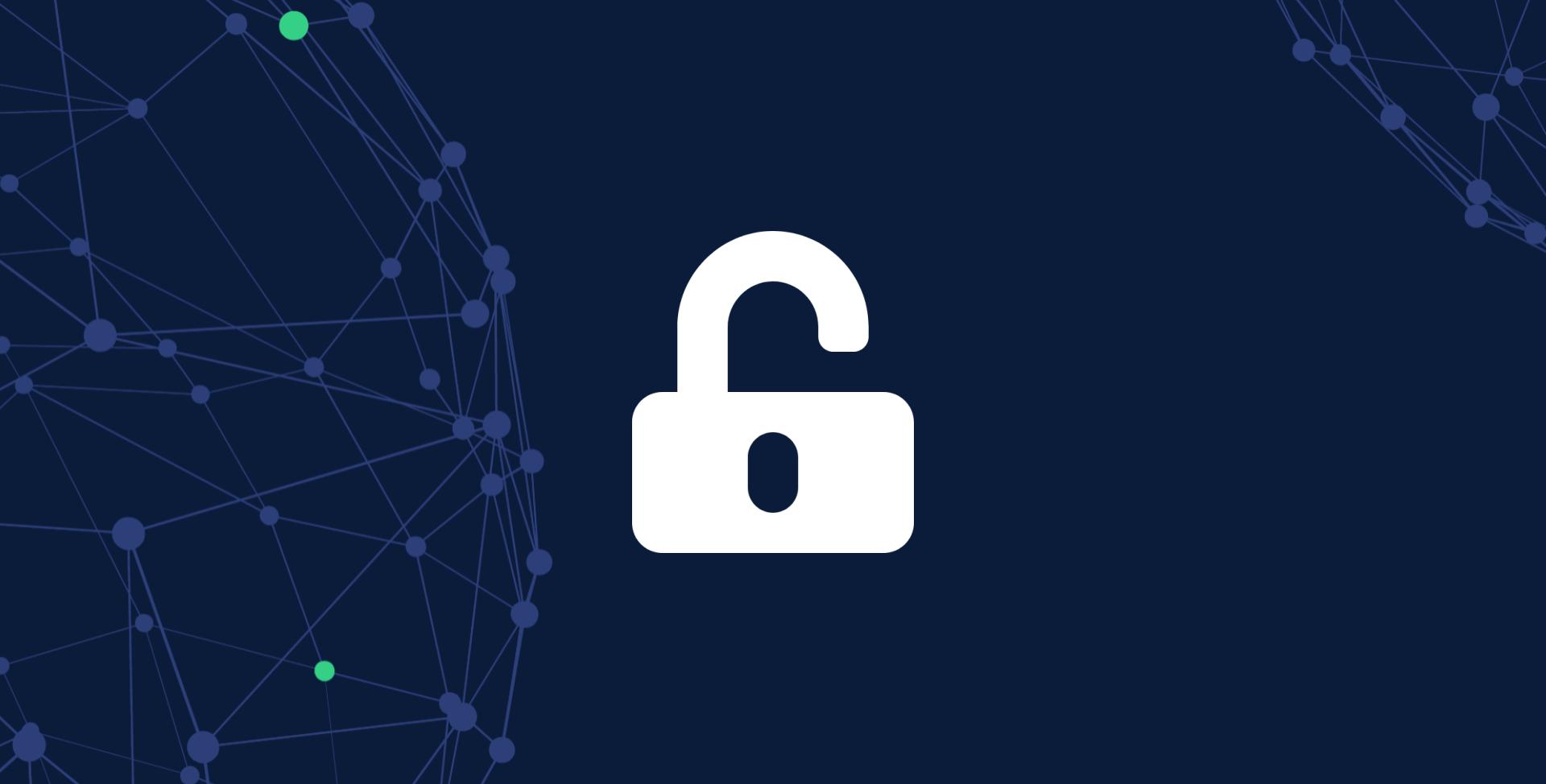
6 Standout Benefits of Private Locations
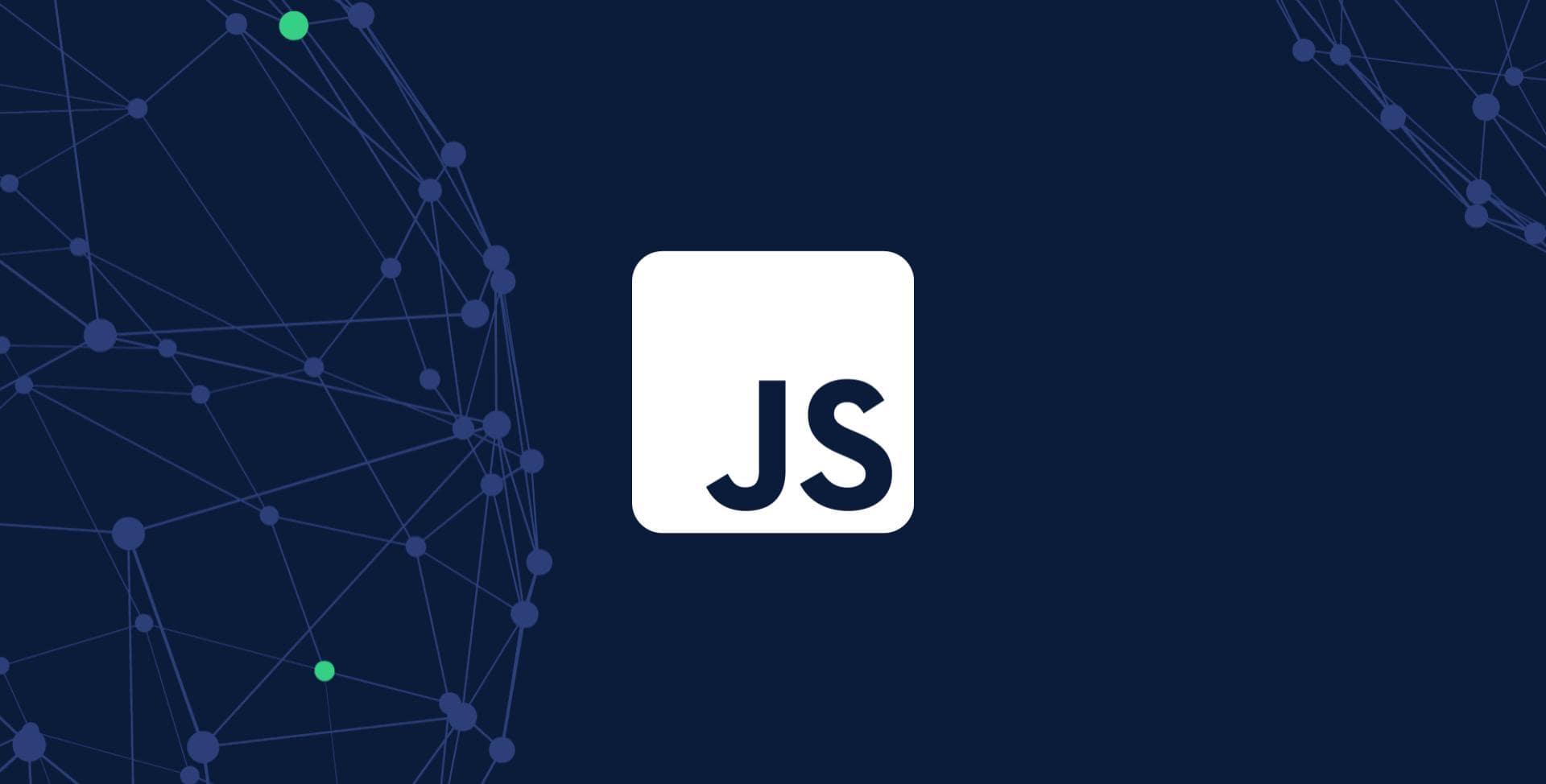