Shift-right testing with load testing metrics
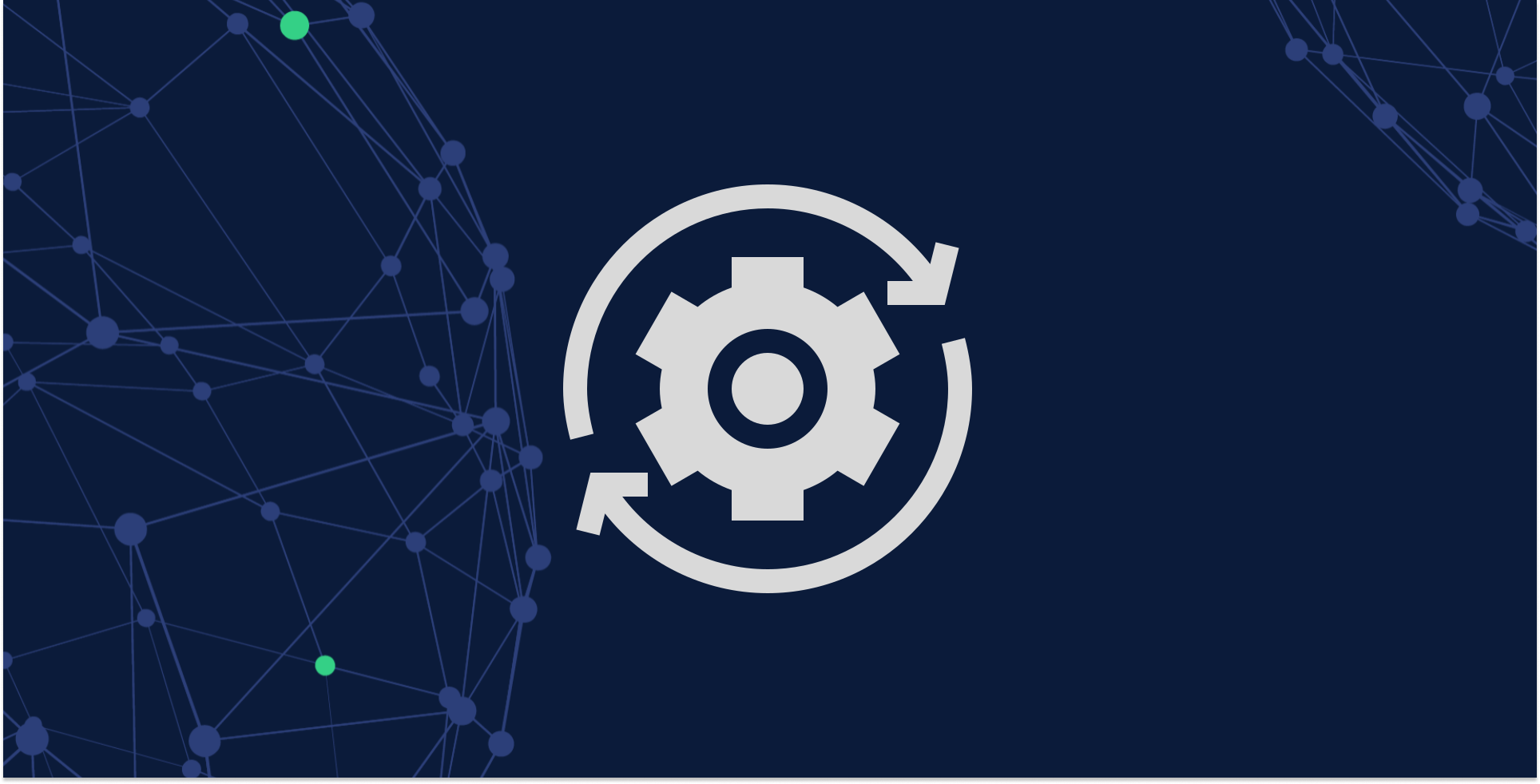
Shift-right testing with load testing metrics
Jul 8, 2025 11:05:59 AM
11
min read
What is GitOps? A developer's guide
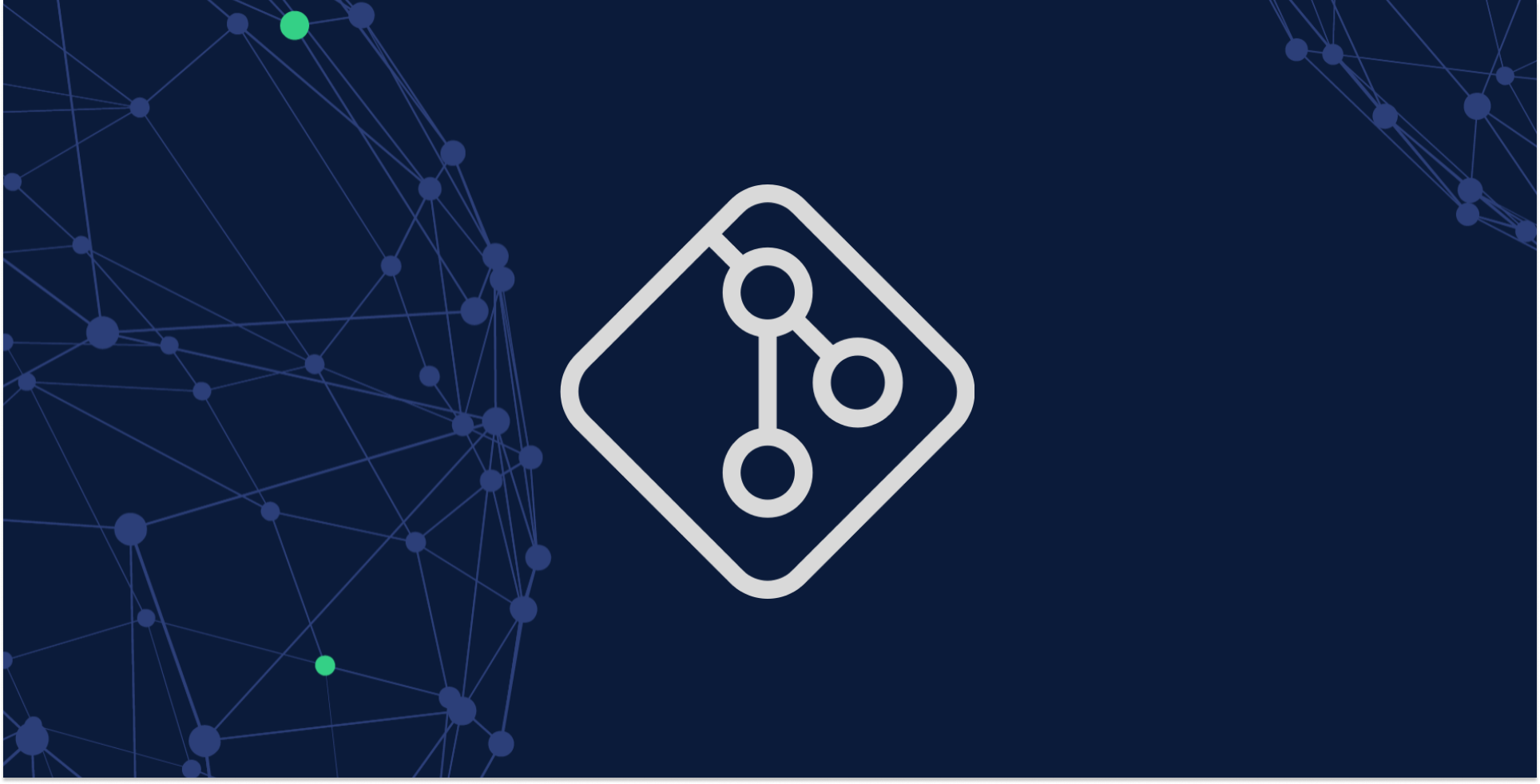
What is GitOps? A developer's guide
Jul 1, 2025 7:22:12 PM
10
min read
10 Performance testing metrics to watch before you ship
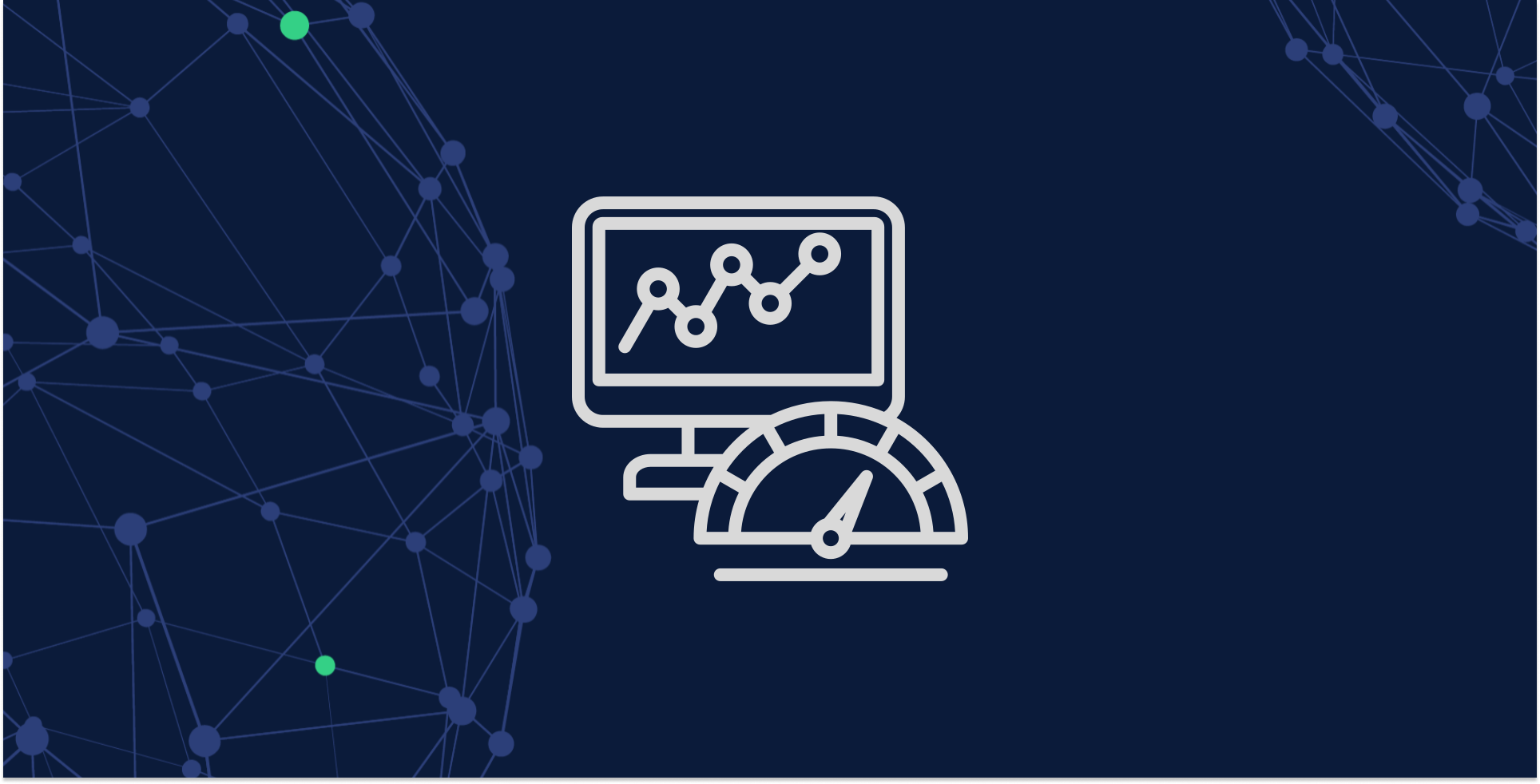
10 Performance testing metrics to watch before you ship
Jun 24, 2025 2:39:32 PM
11
min read
What is the software development lifecycle (SDLC)? Complete guide
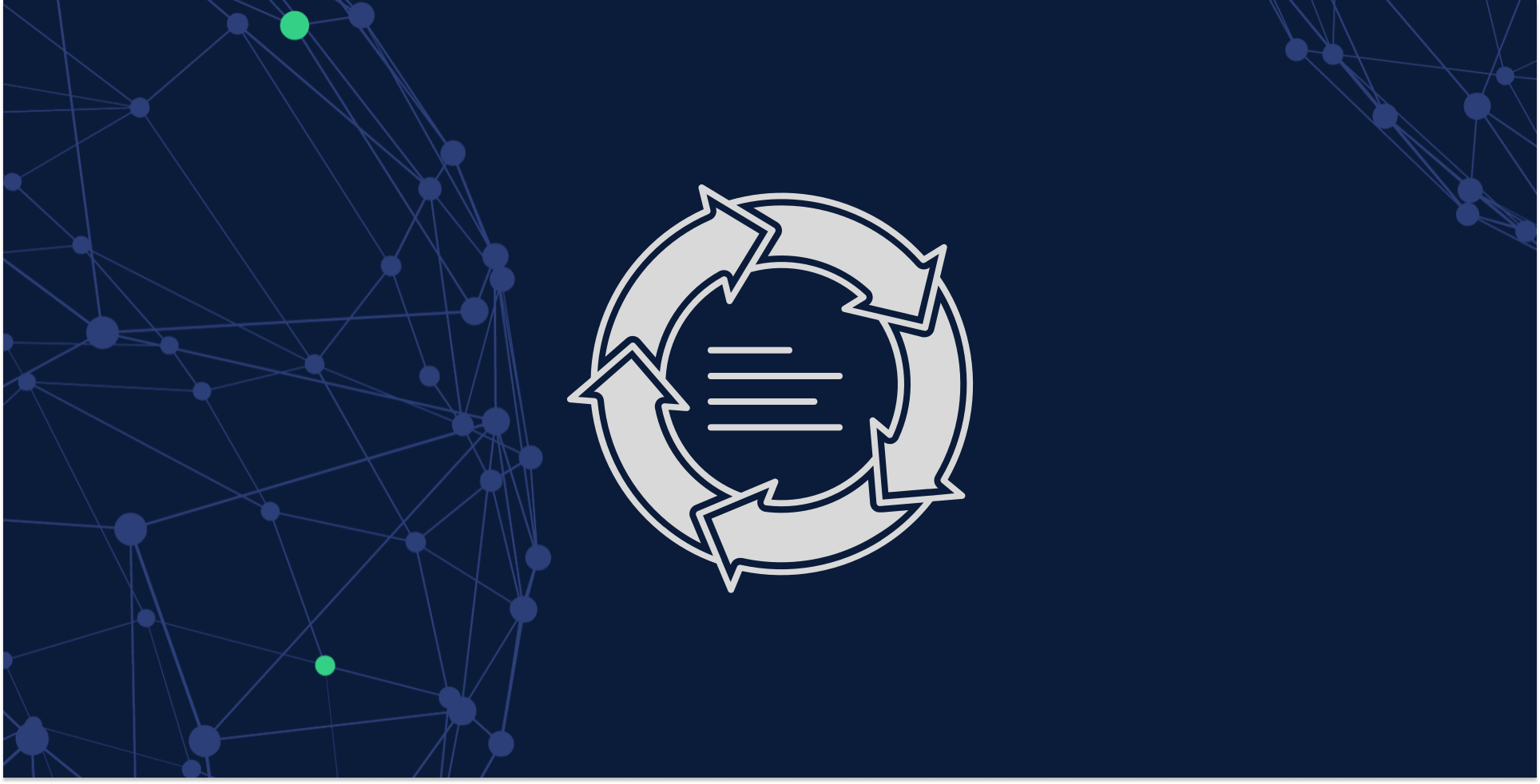
What is the software development lifecycle (SDLC)? Complete guide
Jun 16, 2025 5:49:51 PM
12
min read
Gatling Enterprise now integrates with Datadog
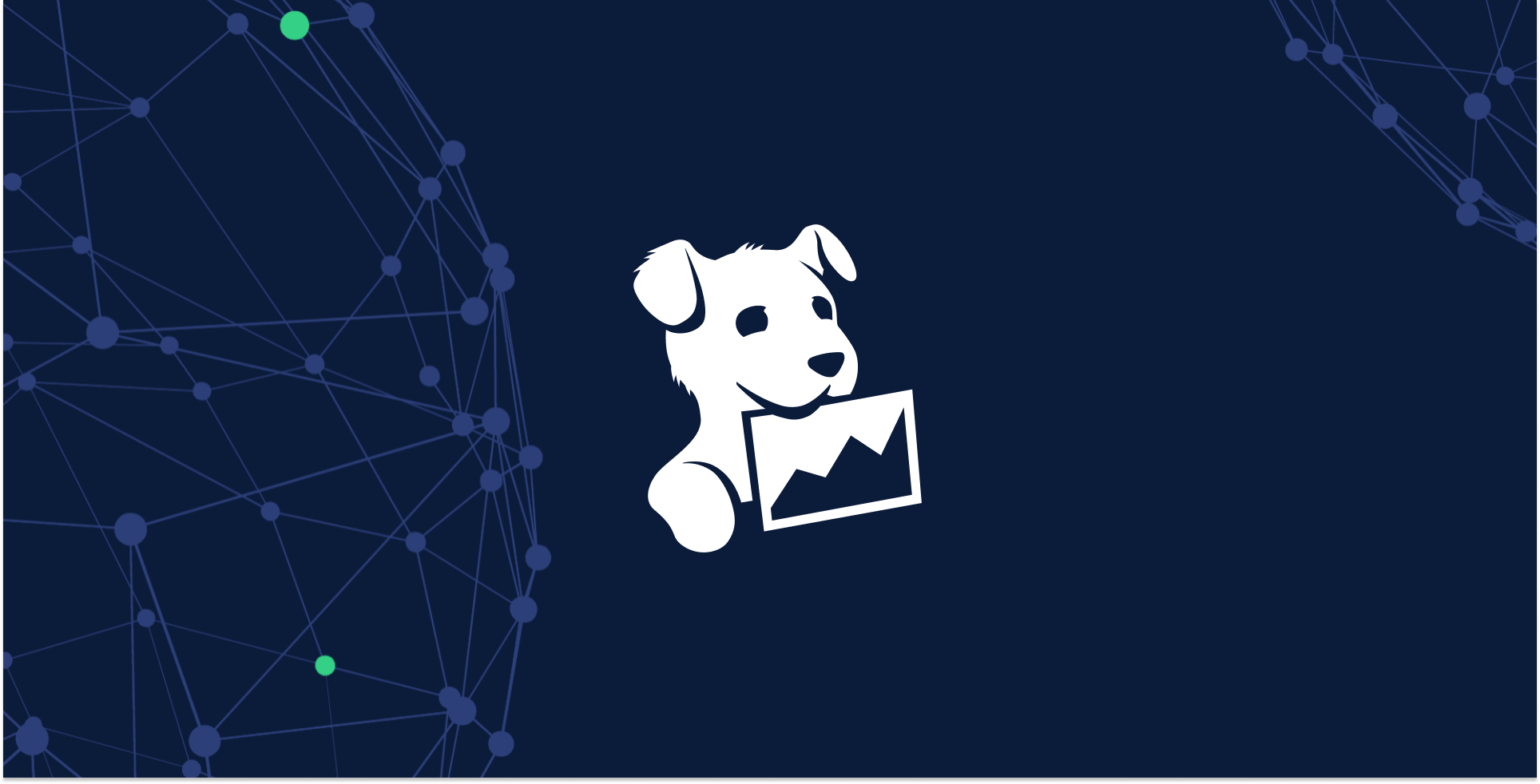
Gatling Enterprise now integrates with Datadog
Jun 12, 2025 12:53:56 PM
1
min read
What is DevSecOps? A guide to secure software development
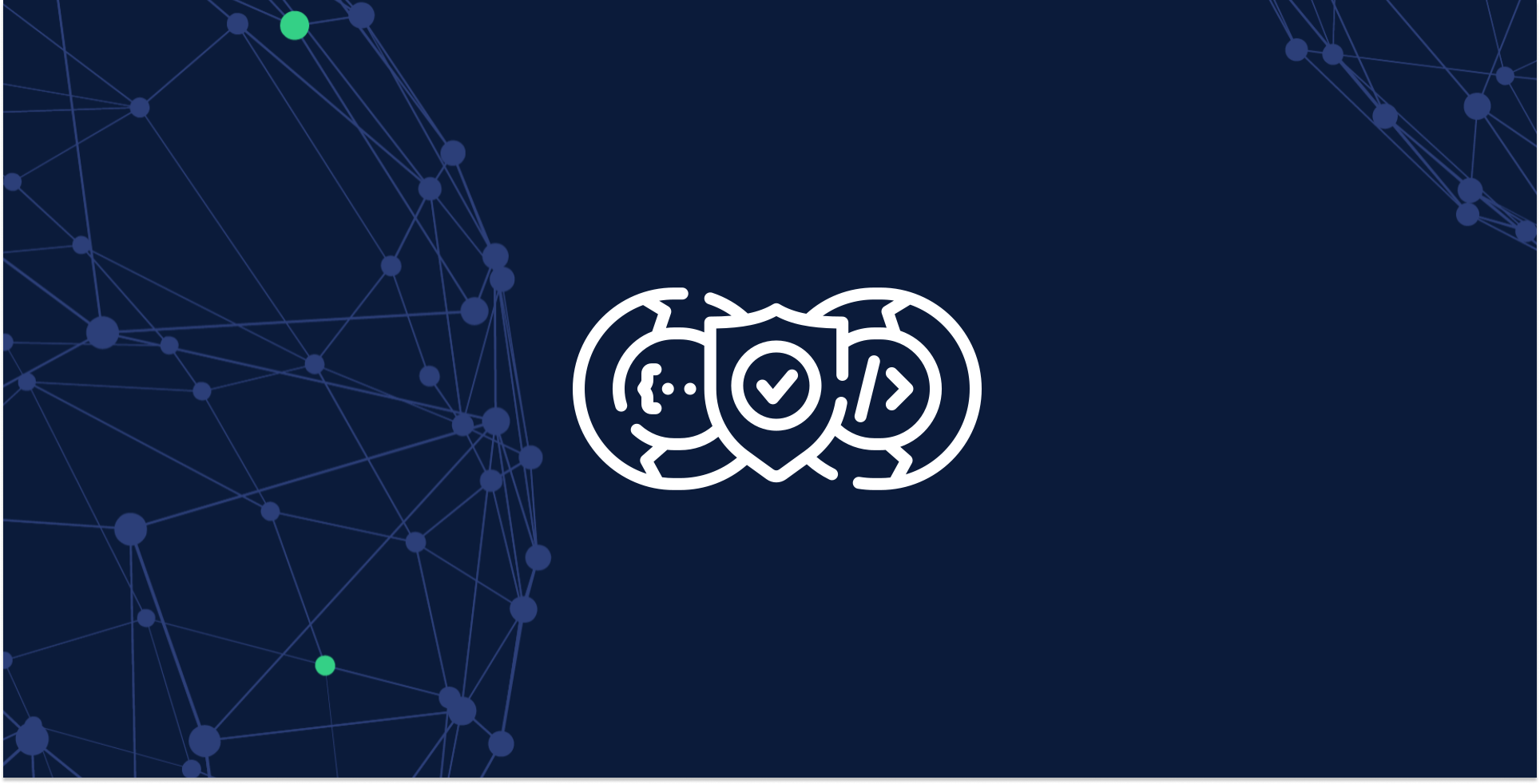
What is DevSecOps? A guide to secure software development
Jun 9, 2025 3:57:24 PM
13
min read
Load testing vs. performance testing: How to start with Gatling
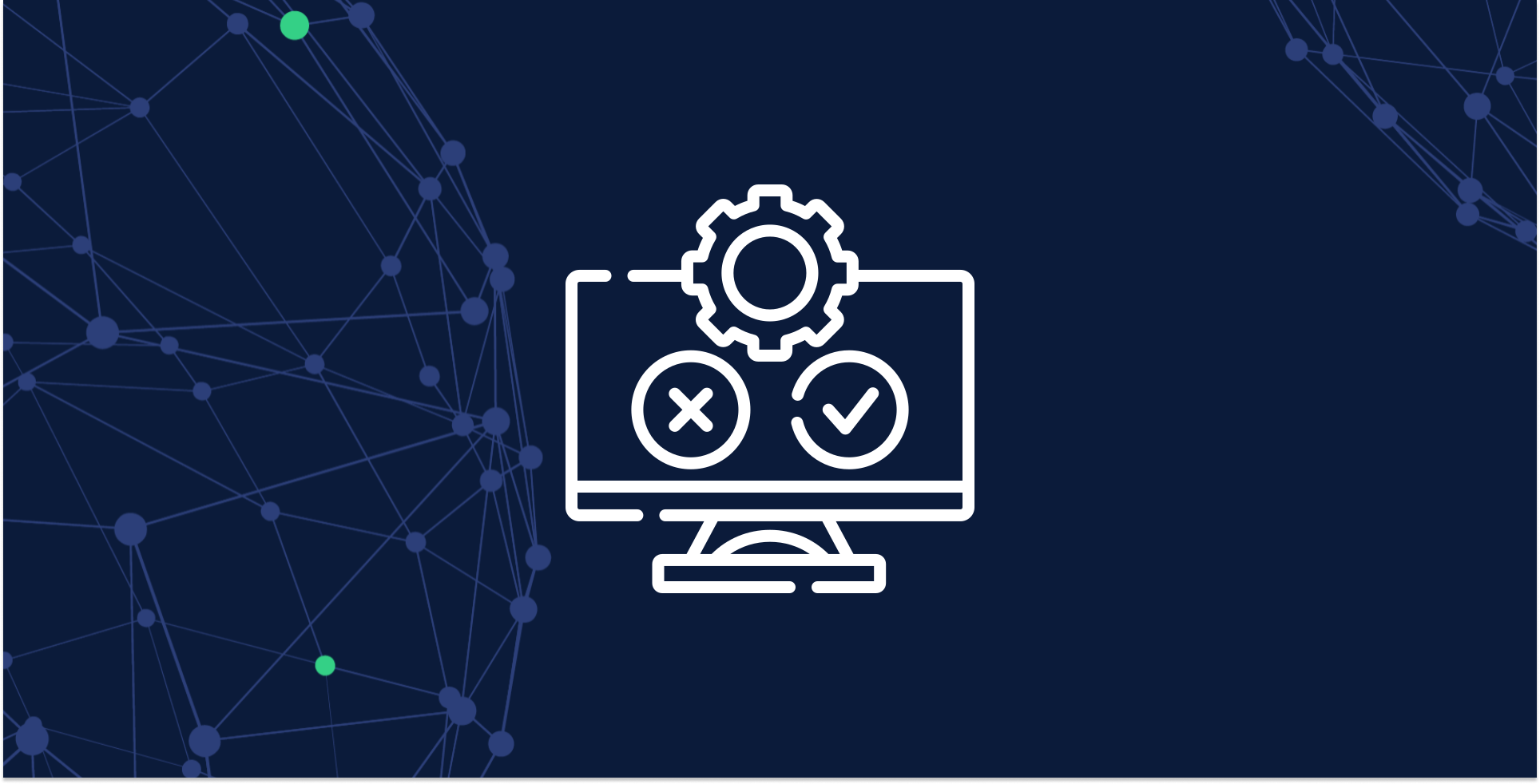
Load testing vs. performance testing: How to start with Gatling
Jun 2, 2025 5:13:46 PM
8
min read
DevOps vs DevSecOps
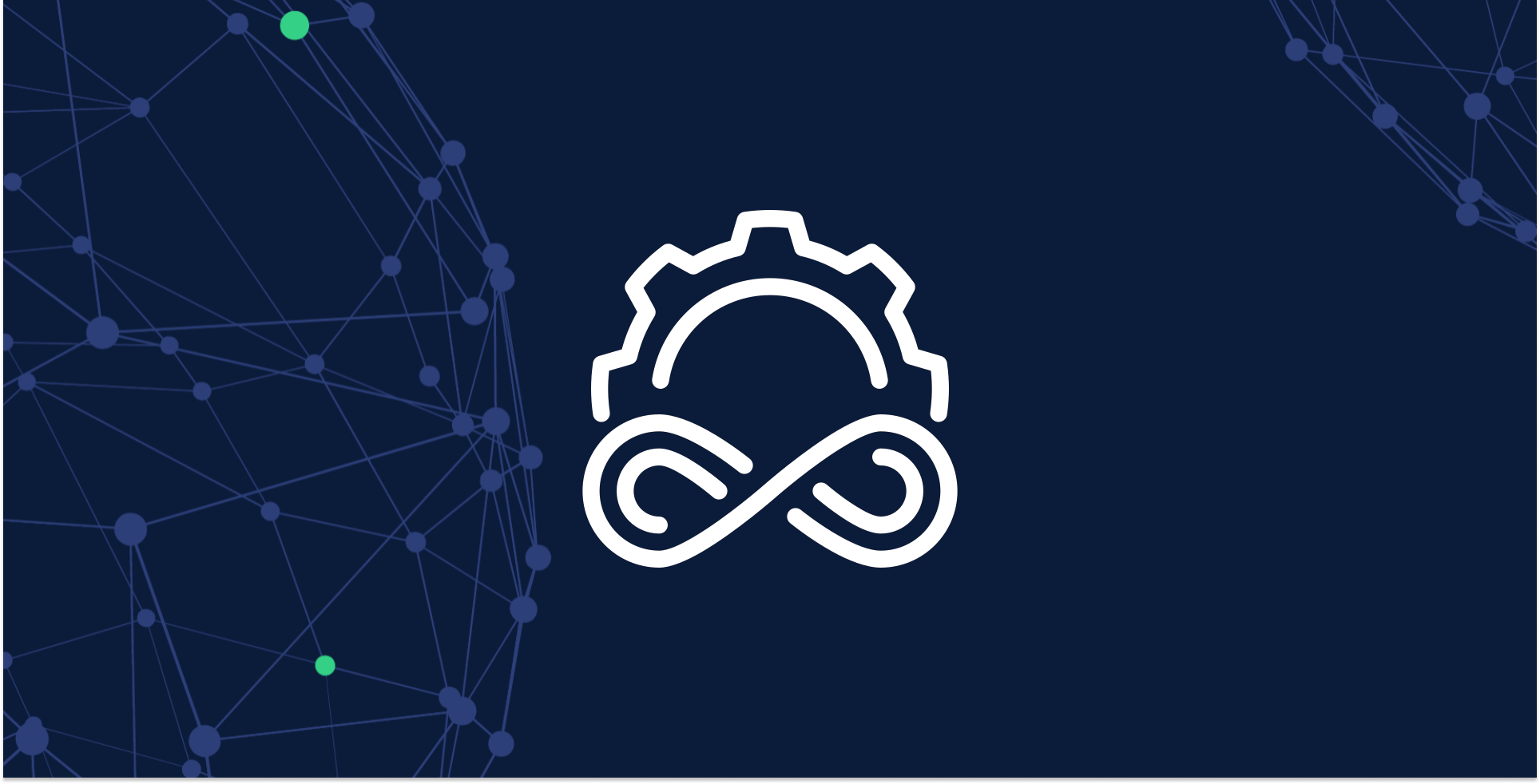
DevOps vs DevSecOps
May 27, 2025 2:02:31 PM
15
min read
Platform Engineering 101: Build Faster, Ship Safer
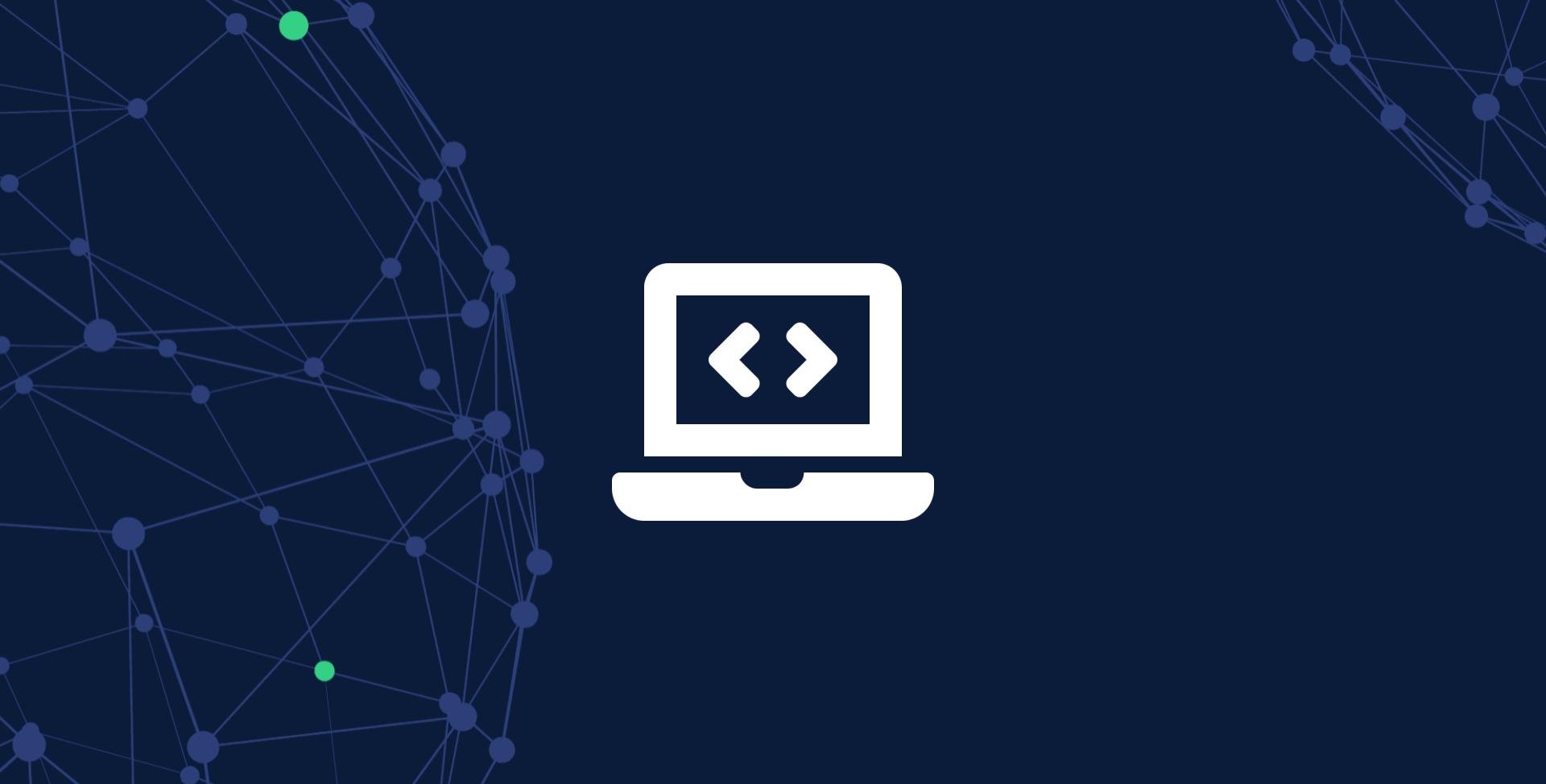
Platform Engineering 101: Build Faster, Ship Safer
May 19, 2025 11:34:21 AM
11
min read