Test faster with enhanced no-code test-building capabilities
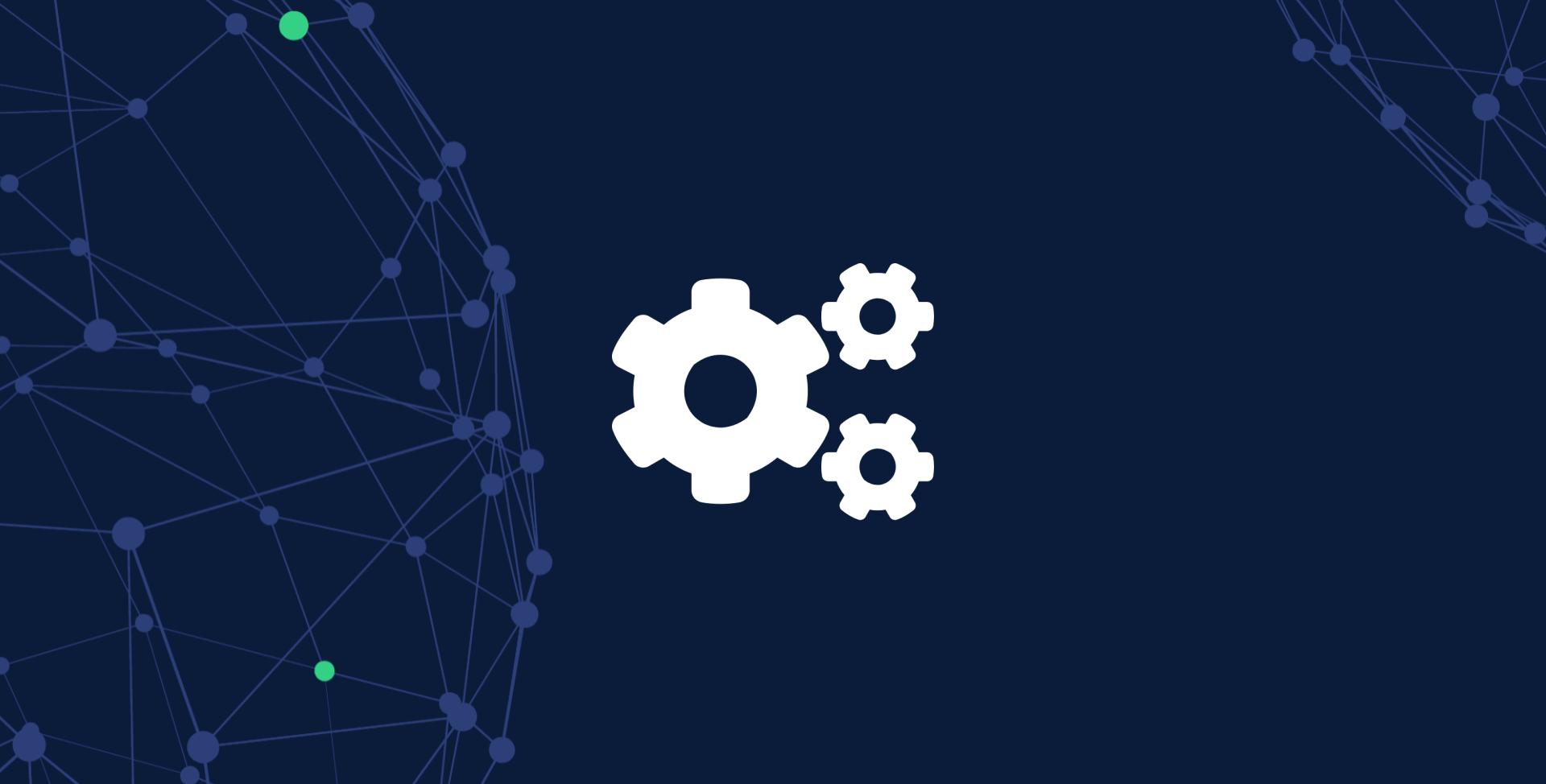
Test faster with enhanced no-code test-building capabilities
Jul 3, 2024 10:59:58 AM
4
min read
Putting SSE to Work: A Load Test LLM API Case Study
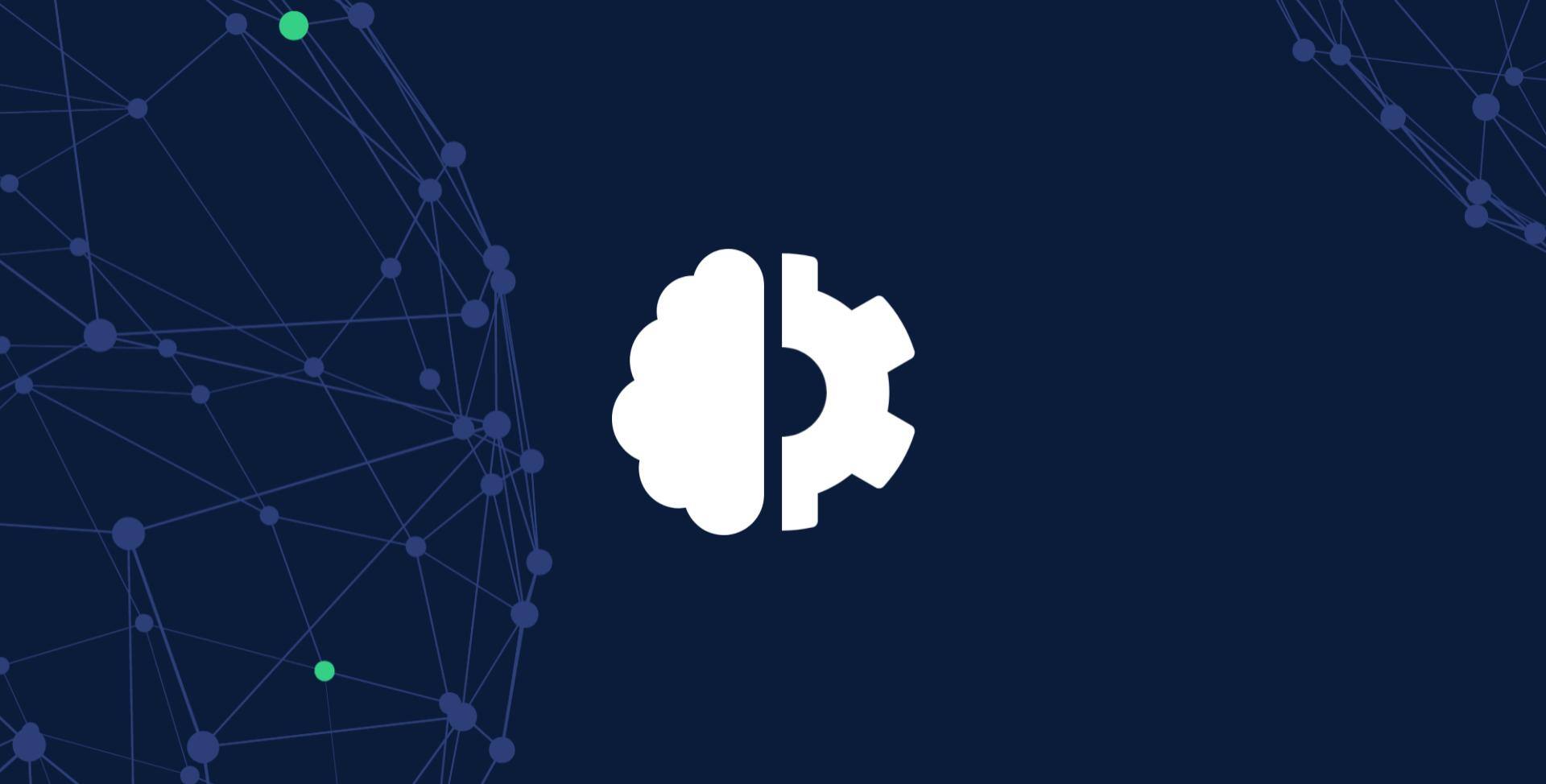
Putting SSE to Work: A Load Test LLM API Case Study
Jun 11, 2024 10:31:41 AM
3
min read
Gatling doubles its availability with a new JavaScript SDK
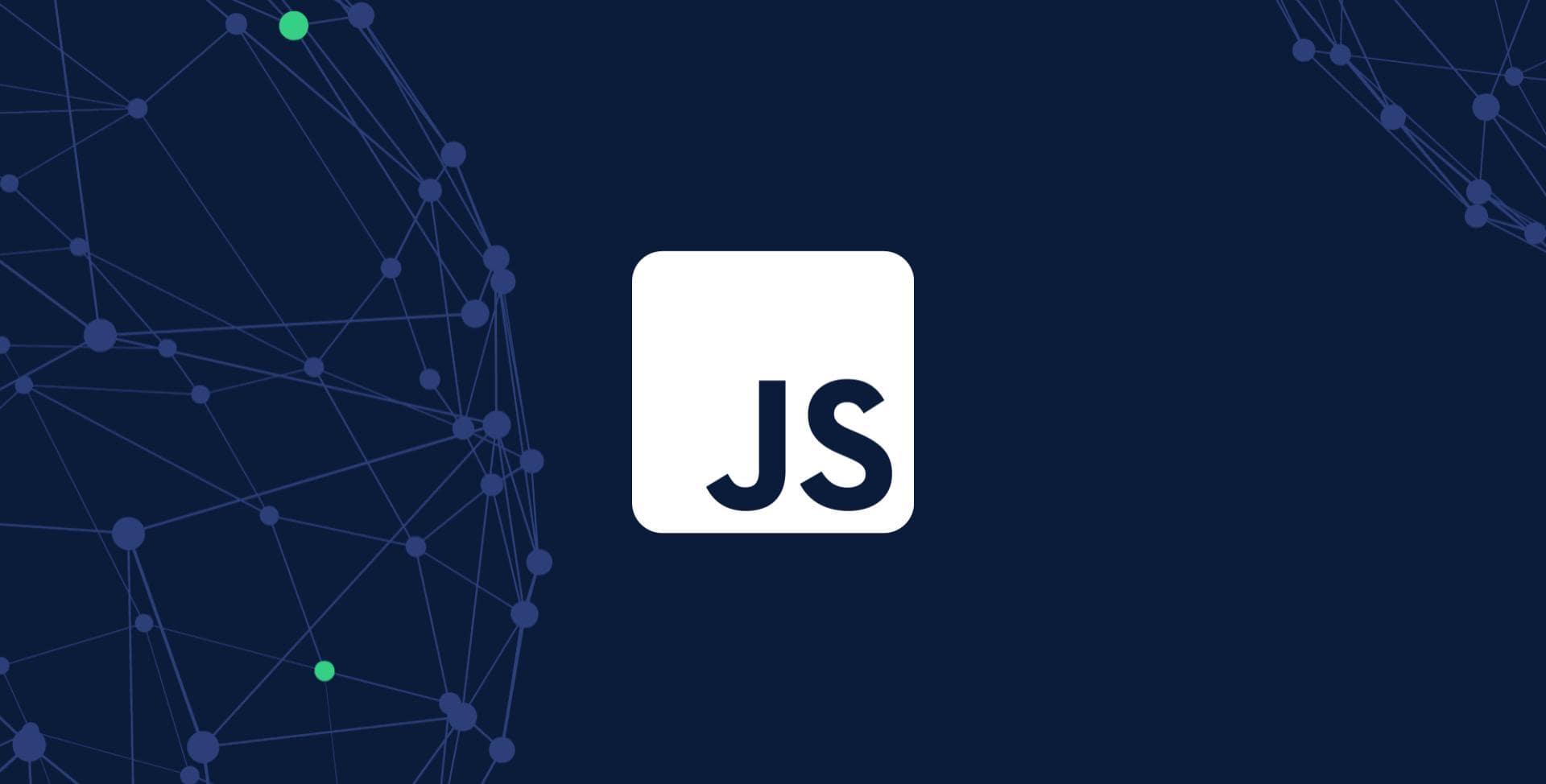
Gatling doubles its availability with a new JavaScript SDK
May 23, 2024 2:56:30 PM
4
min read
Gatling releases Configuration as Code for Enterprise users
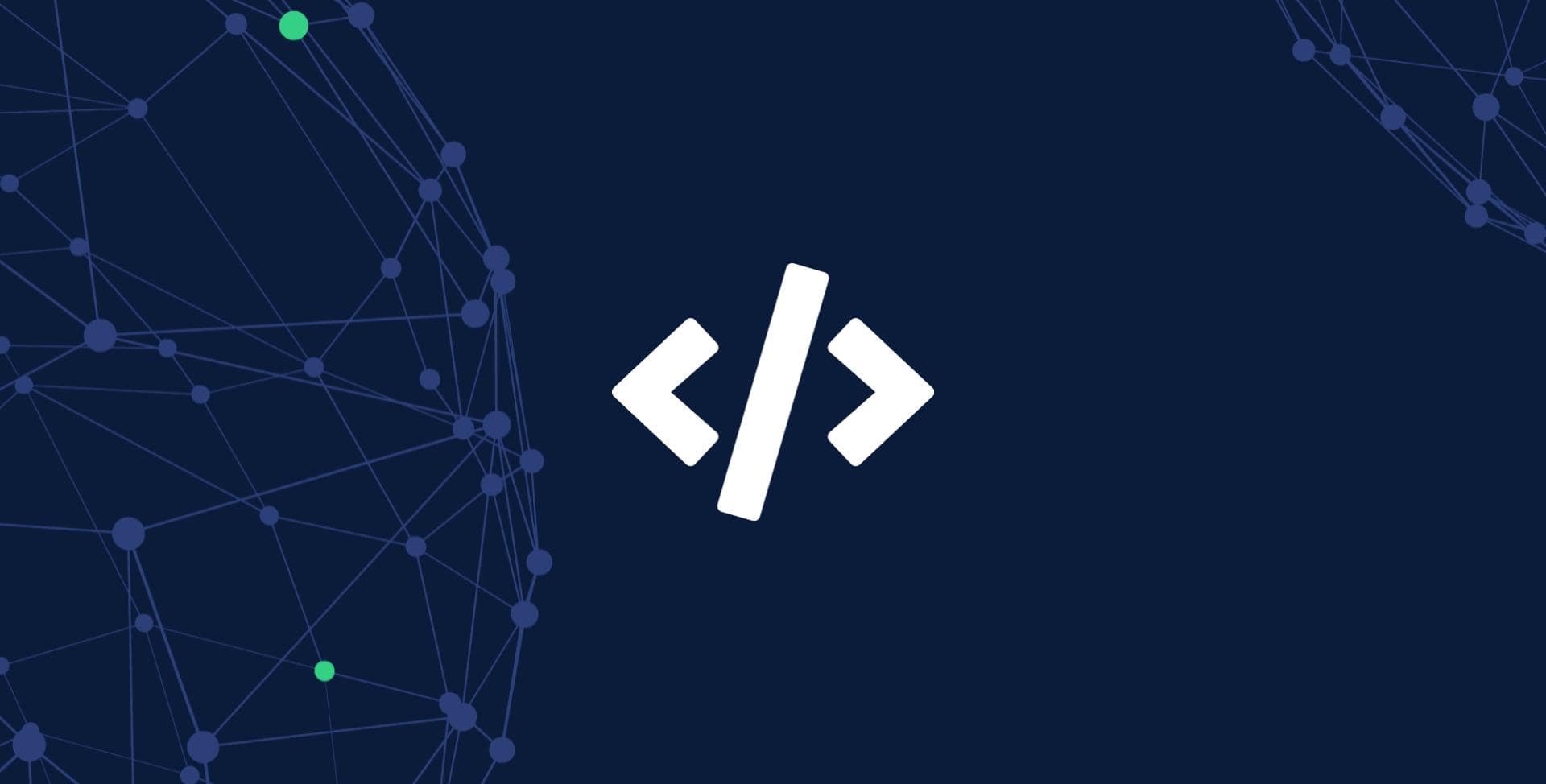
Gatling releases Configuration as Code for Enterprise users
Apr 30, 2024 9:15:00 AM
3
min read
Gatling 3.11 is now available
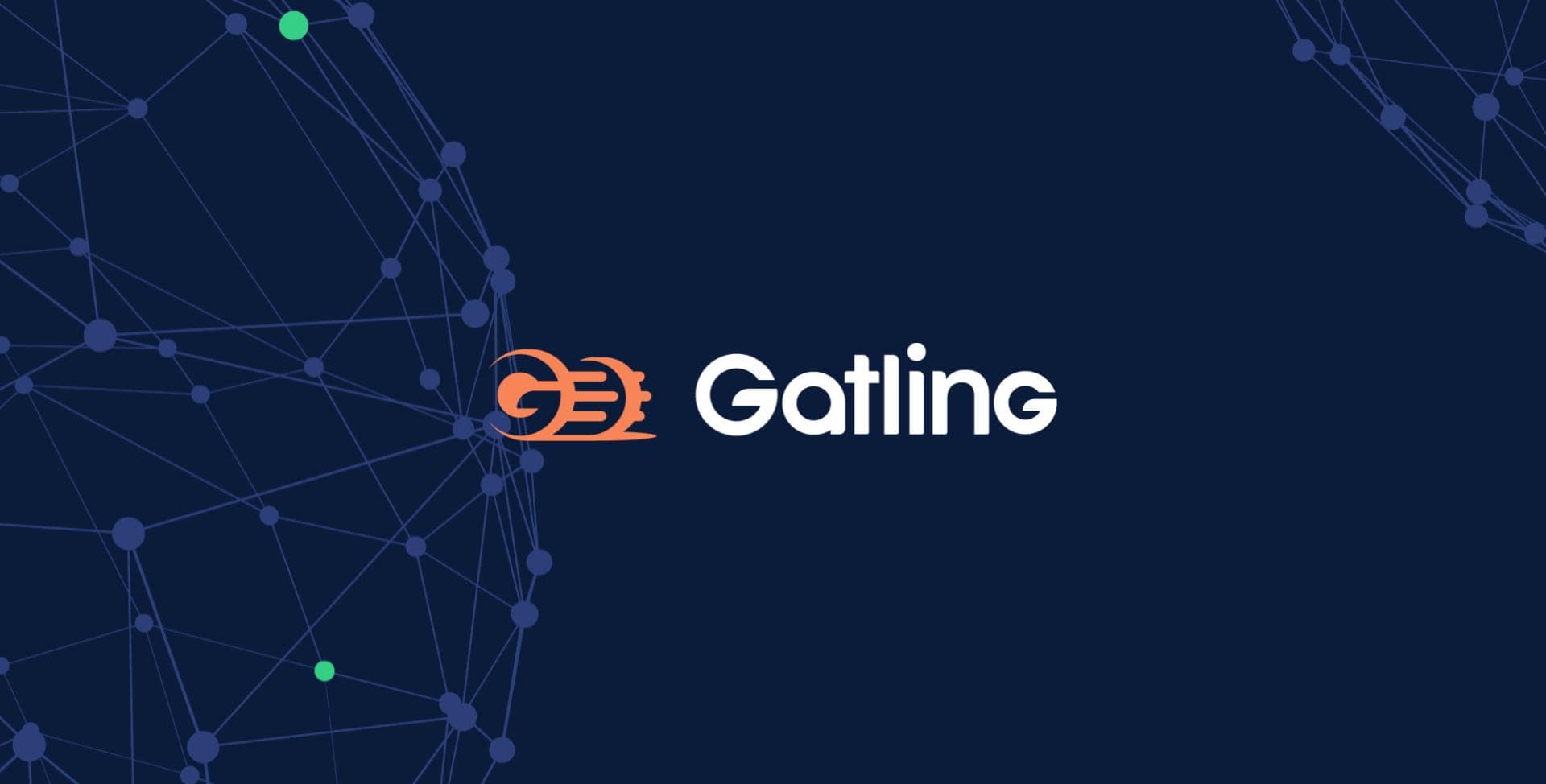
Gatling 3.11 is now available
Apr 25, 2024 1:18:10 PM
3
min read
MQTT Performance Testing using Gatling
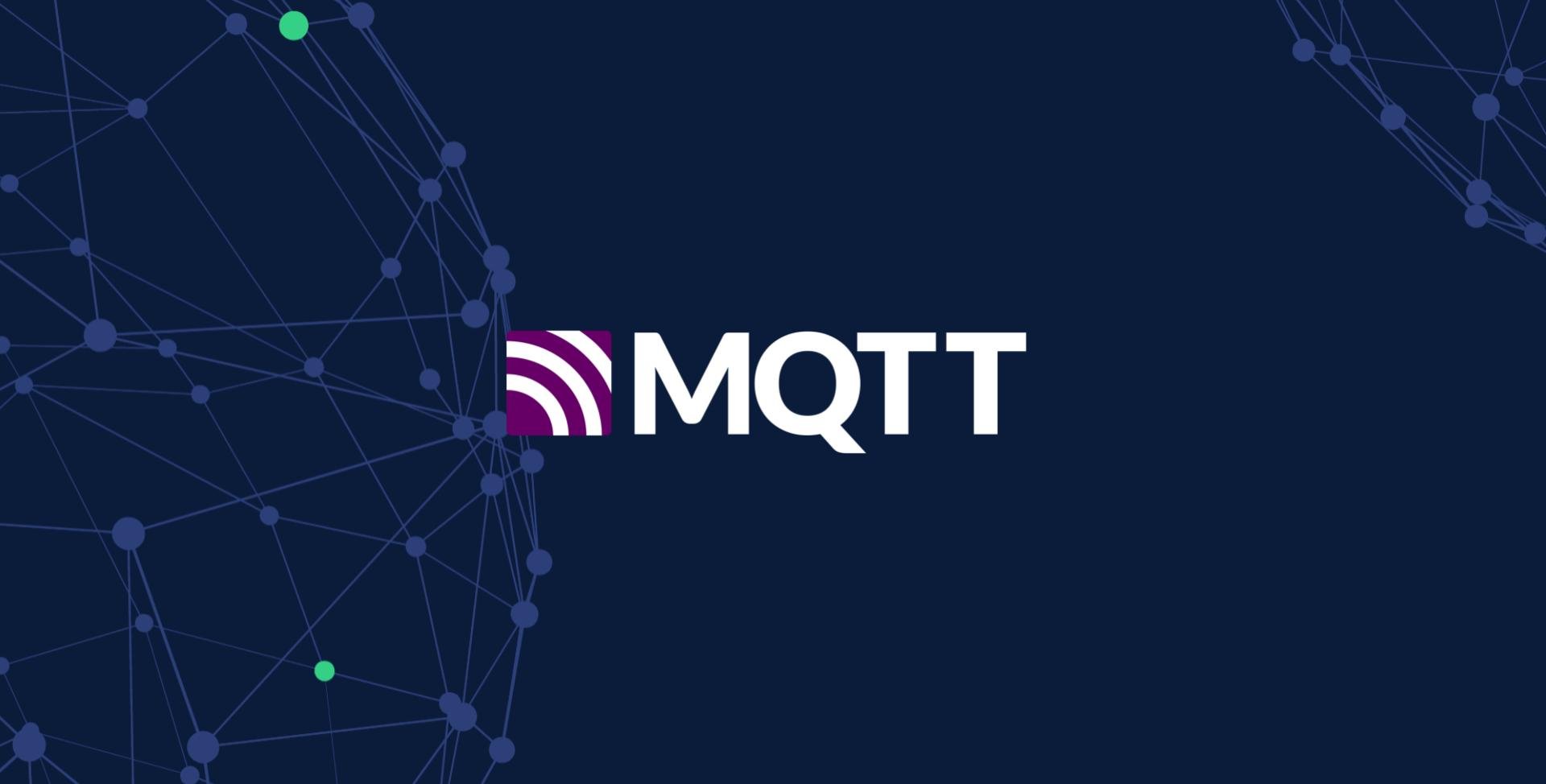
MQTT Performance Testing using Gatling
Mar 28, 2024 11:00:00 AM
6
min read
Transport Layer Security (TLS) and application performance
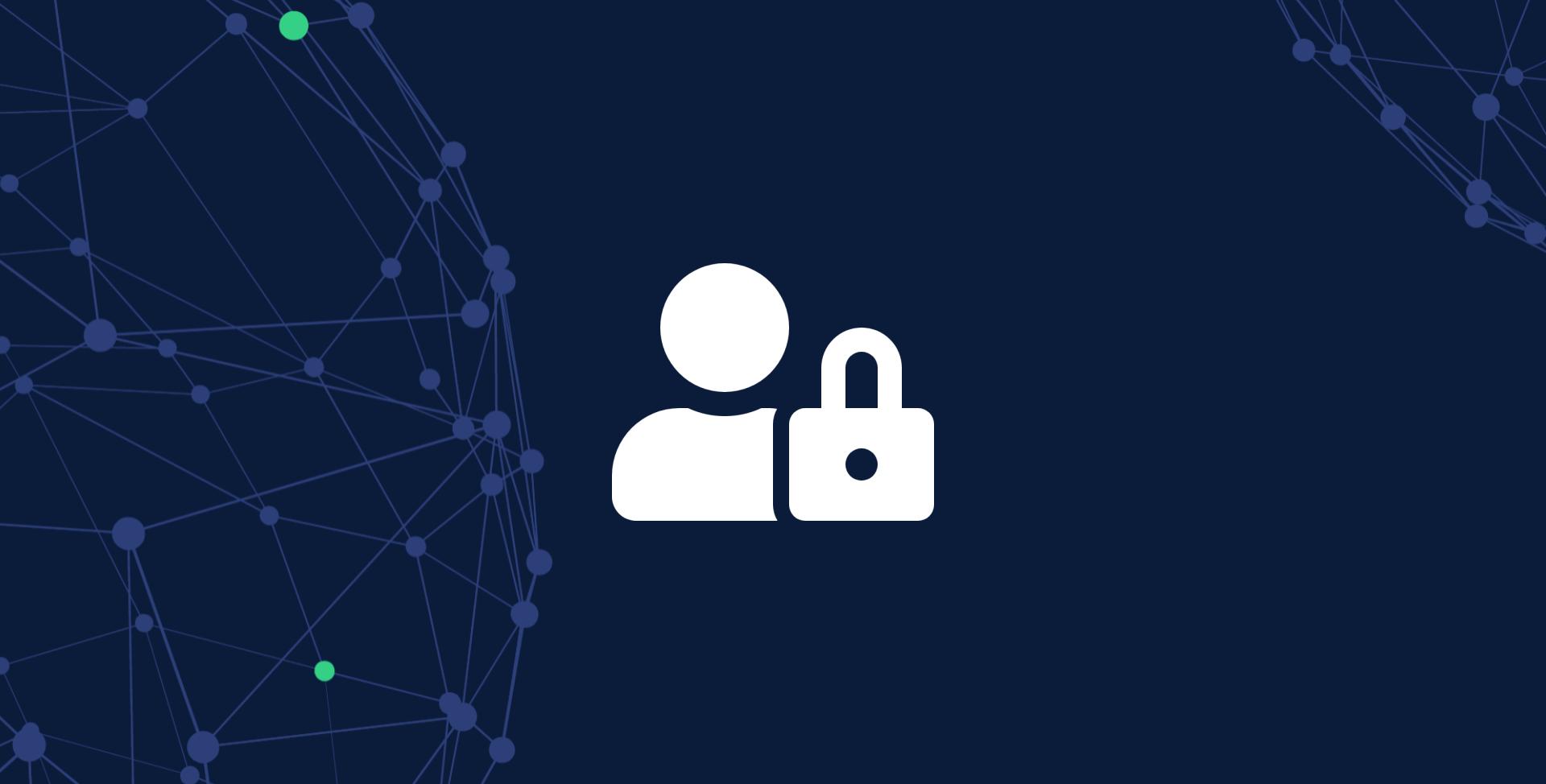
Transport Layer Security (TLS) and application performance
Feb 6, 2024 9:58:00 AM
5
min read
Gatling introduces a gRPC plugin for load testing
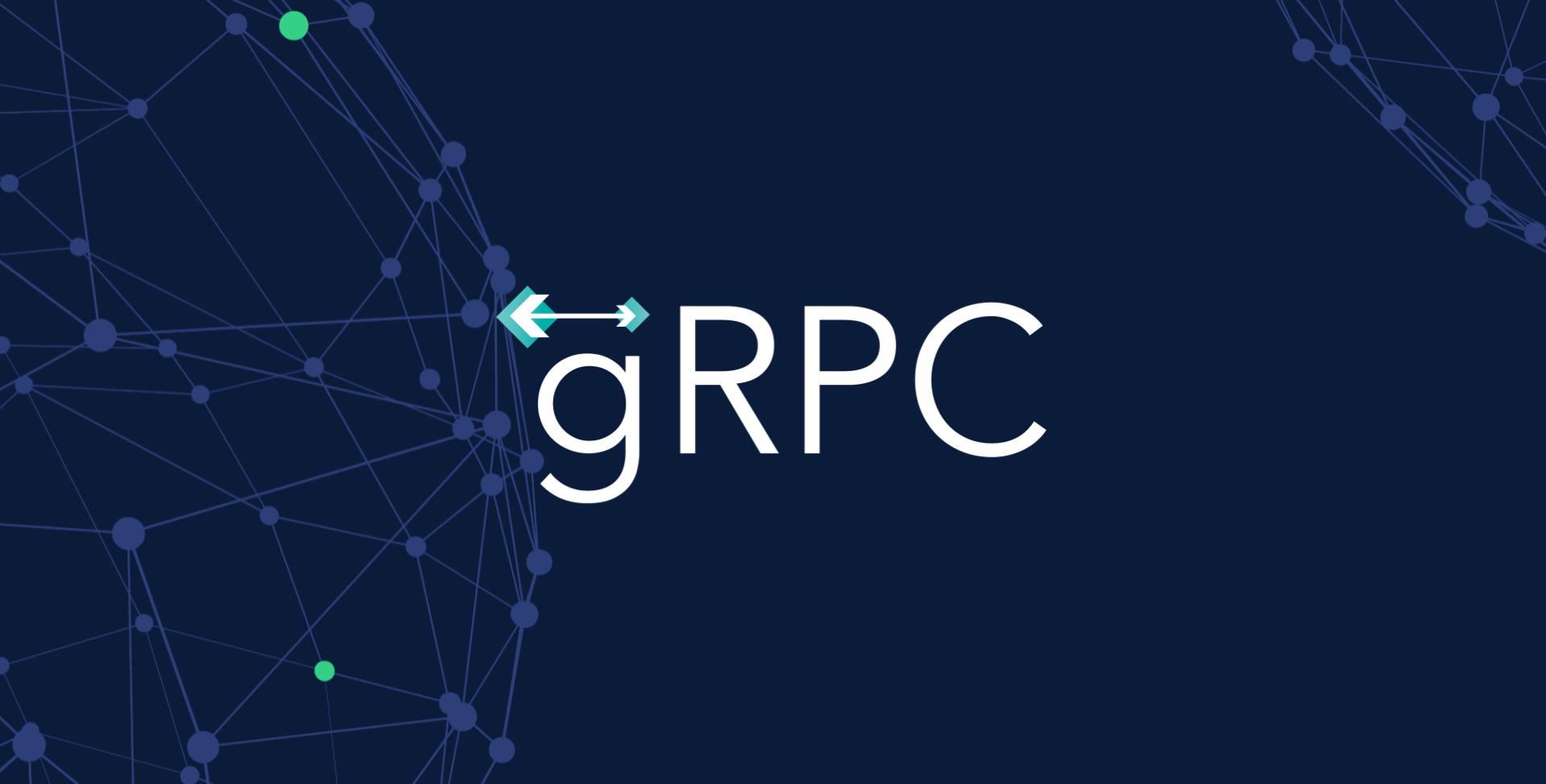
Gatling introduces a gRPC plugin for load testing
Jan 30, 2024 10:23:00 AM
3
min read
Shift left Testing What, Why, and How to Get Started
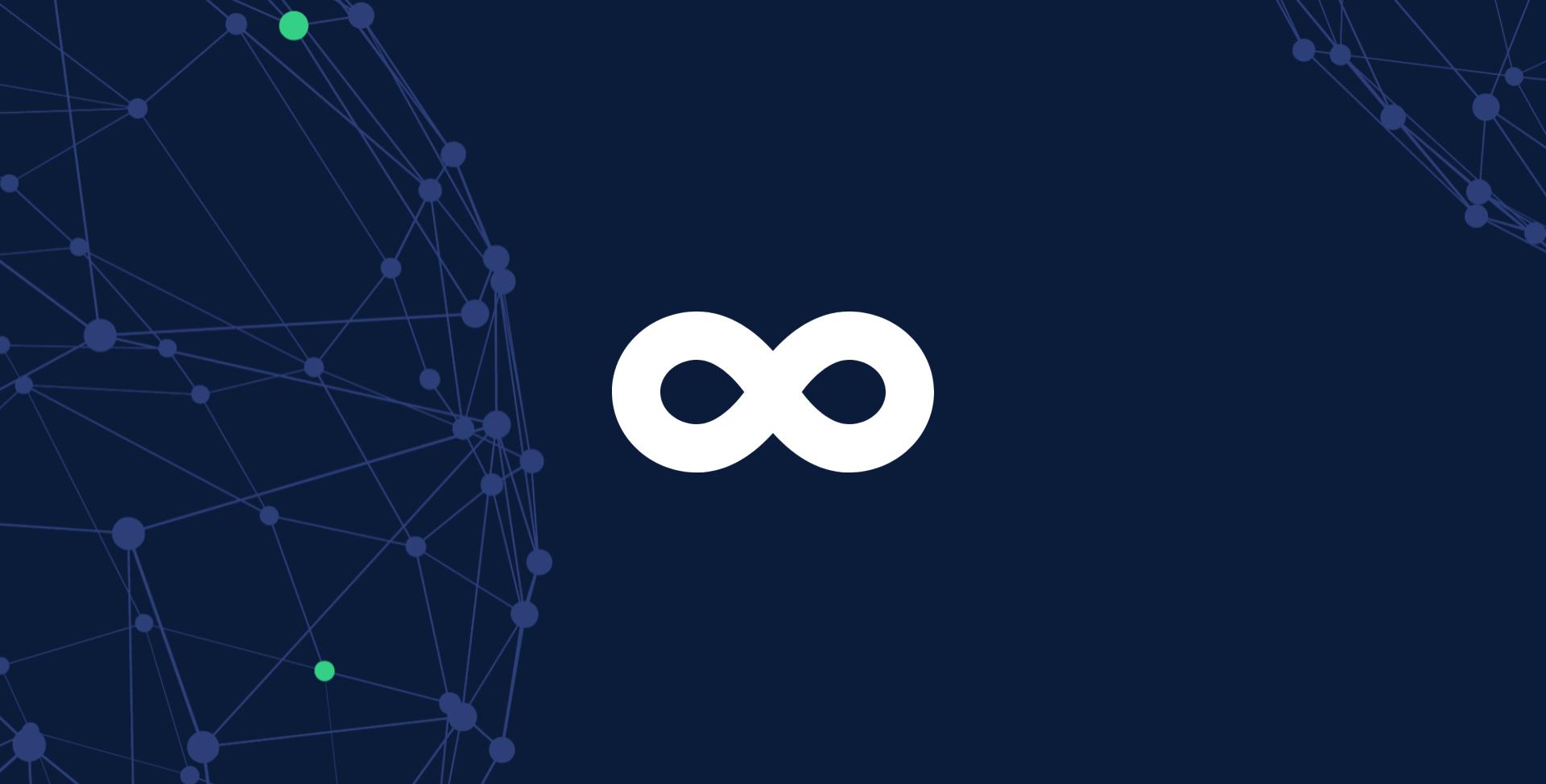
Shift left Testing What, Why, and How to Get Started
Jan 22, 2024 5:24:00 PM
5
min read