How to load test MQTT applications with Gatling
MQTT is the go-to protocol for real-time, lightweight communication across millions of devices. From smart homes to industrial IoT, MQTT powers systems where low latency and reliability are non-negotiable.
But here’s the catch: when your app scales, performance bottlenecks often surface where you least expect them—impacting both device-to-cloud communication and user experience.
So how do you make sure your MQTT infrastructure performs at scale?
What is MQTT?
MQTT (Message Queuing Telemetry Transport) is a lightweight publish-subscribe protocol designed for real-time communication between devices, services, and applications. Originally developed for oil pipeline monitoring, it’s now the backbone of IoT ecosystems, messaging platforms, and automation systems.
MQTT is ideal for scenarios where:
-
Network bandwidth is limited
-
Devices frequently disconnect and reconnect
-
Data must be sent and received with minimal overhead
MQTT protocol components
-
MQTT clients: Publishers and subscribers that send or receive data
-
MQTT broker: A central service that routes messages to subscribers based on topics
-
Topic: A string that defines message routing rules (like
home/temperature
) -
Retained message: A broker feature that stores the last message sent to a topic for new subscribers
-
QoS levels: Control delivery guarantees across the network
-
Authentication and TLS encryption: Essential for secure deployments
MQTT is widely supported by open-source software, comes in multiple MQTT versions (including 3.1.1 and 5.0), and supports JSON, binary, and plain text payloads. It’s used for sending data, sharing information, and coordinating actions across thousands of devices.
MQTT protocols need specialized testing
Most load testing tools are built for HTTP APIs and traditional web traffic. MQTT, being a stateful, event-driven protocol, behaves differently:
- Persistent connections must be handled across thousands of clients
- Topic-based message distribution adds architectural complexity
- QoS levels, retained messages, and will messages introduce variables that impact performance
Without a purpose-built approach to MQTT load testing, teams are often left guessing:
- Can our broker handle thousands of concurrent connections?
- Will latency spike under high throughput?
- Are messages reliably delivered under stress?
Unfortunately, these questions are usually answered too late—in production.
Avoiding downtime in IoT, messaging, and Edge applications
Whether you’re building connected devices, live chat, or telemetry platforms, MQTT performance directly affects:
- Device responsiveness and uptime
- Data accuracy and loss prevention
- Customer experience and SLA compliance
Testing MQTT systems at scale isn’t just about robustness—it’s about reliability, cost efficiency, and avoiding production failures in high-stakes environments.
Companies that skip performance testing for MQTT often end up over-provisioning infrastructure or, worse, firefighting stability issues during customer-facing events.
Gatling + MQTT plugin
Gatling is widely known for its powerful load testing engine and test-as-code flexibility. With the MQTT plugin, teams can now simulate thousands of real-time MQTT clients—publishing, subscribing, and managing connections—at scale.
Key features for MQTT testing with Gatling
-
Test-as-code model with full language support (Java, Scala, Kotlin, TypeScript)
-
Automation-ready via CI/CD integrations
-
Custom injection profiles to model ramp-up, soak, and spike tests
-
Real-time dashboards, historical comparisons, and regression alerts
-
Support for open source tools and third-party brokers
Whether you're testing on-premises deployments, hybrid cloud services, or distributed IoT infrastructures, Gatling makes it easy to stress your system from 0 to thousands of concurrent connections.
Follow our full tutorial to start building and running MQTT tests with Gatling:
Share this
You May Also Like
These Related Articles
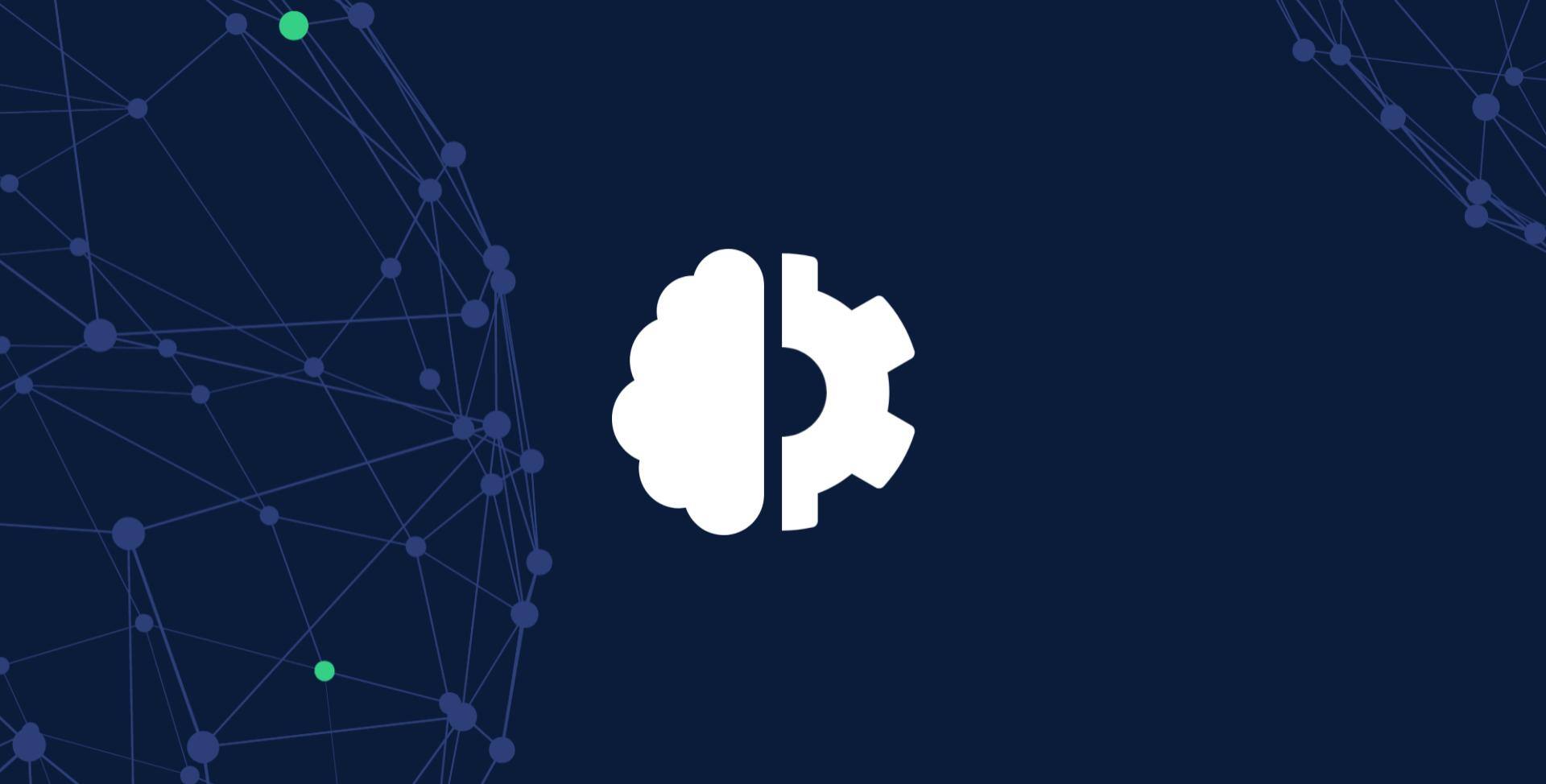
Is Your LLM API ready for real-world load?
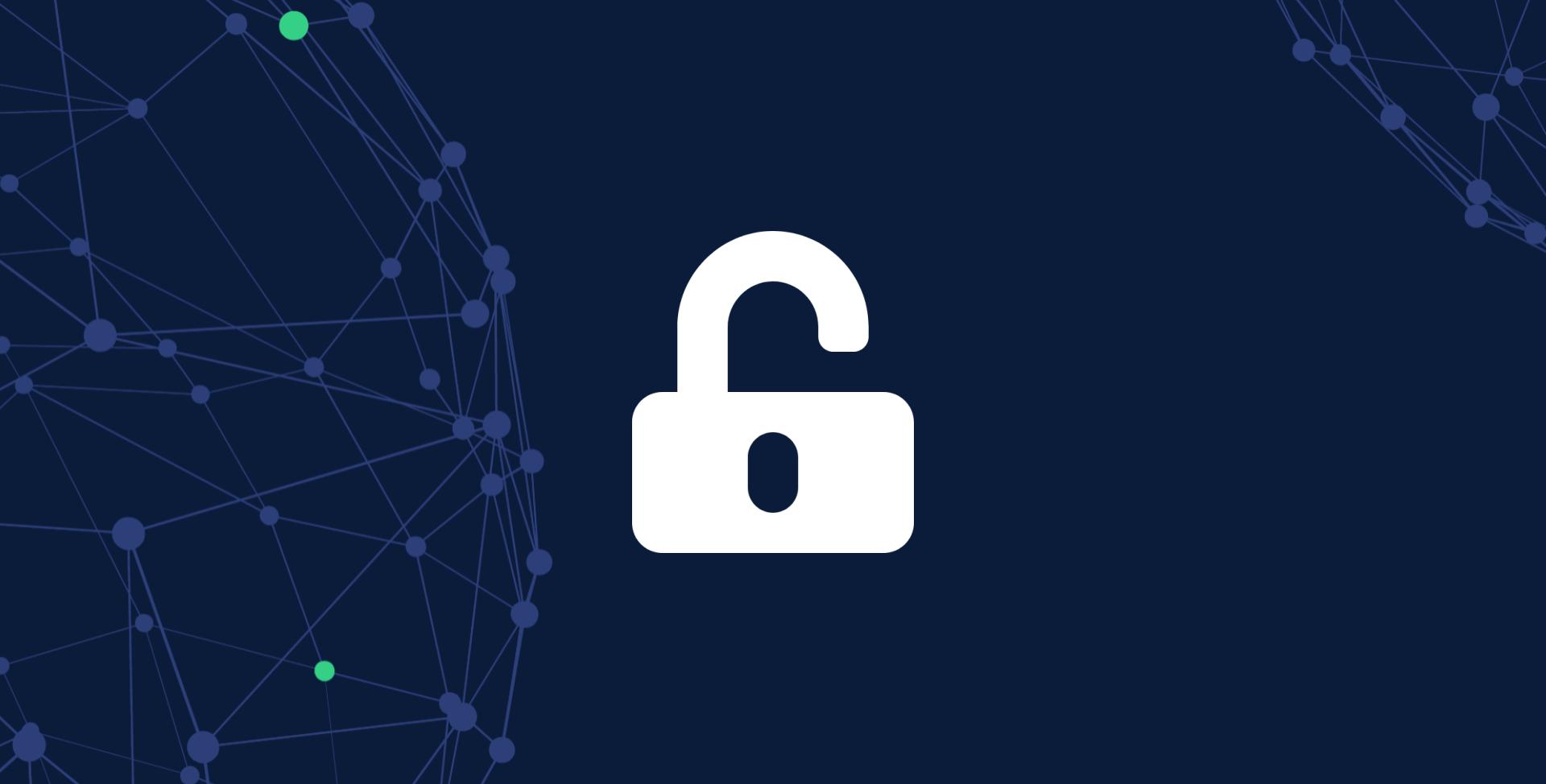
6 Standout Benefits of Private Locations
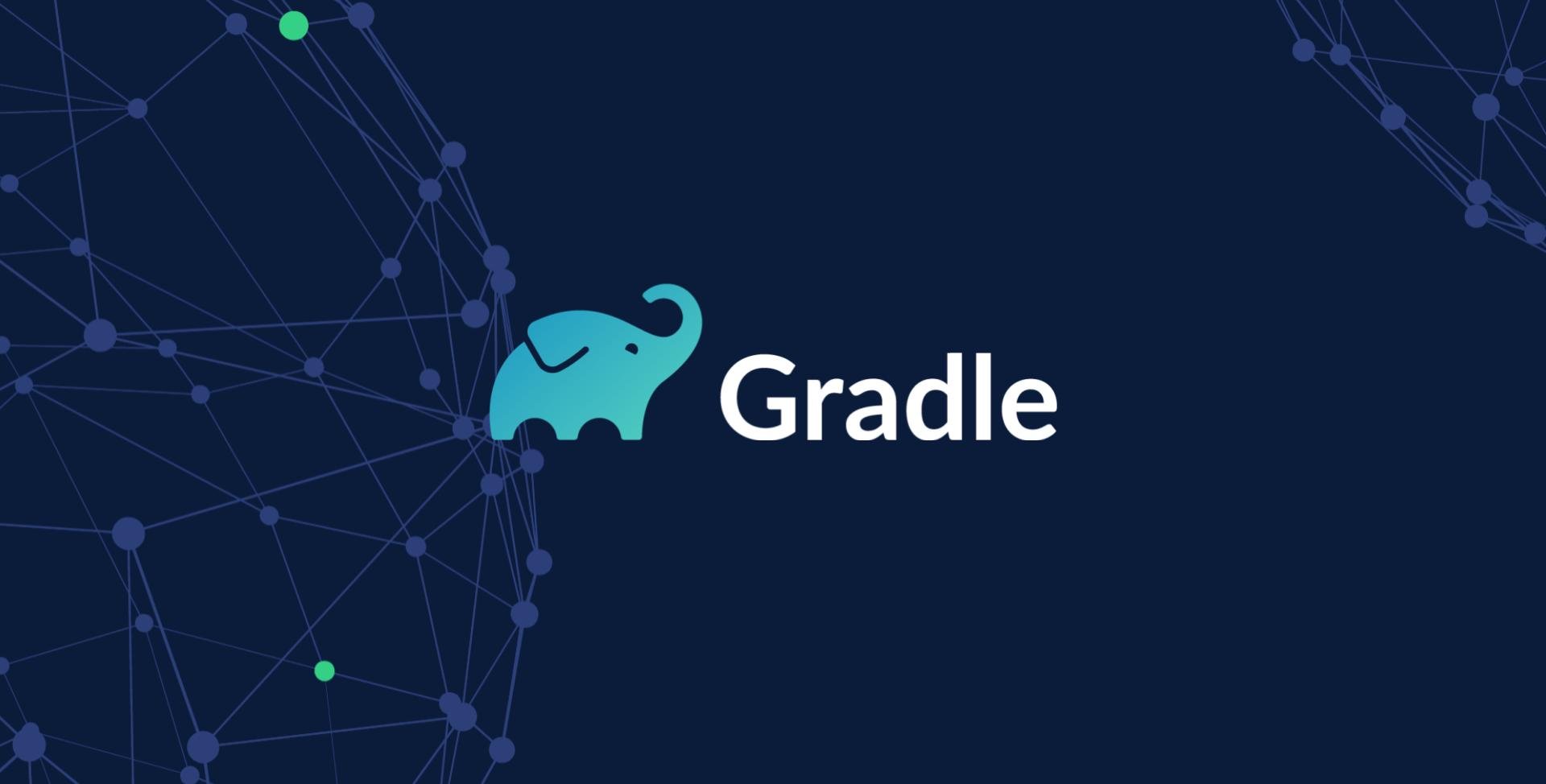